~ 5 min read
Is Node.js Secure?
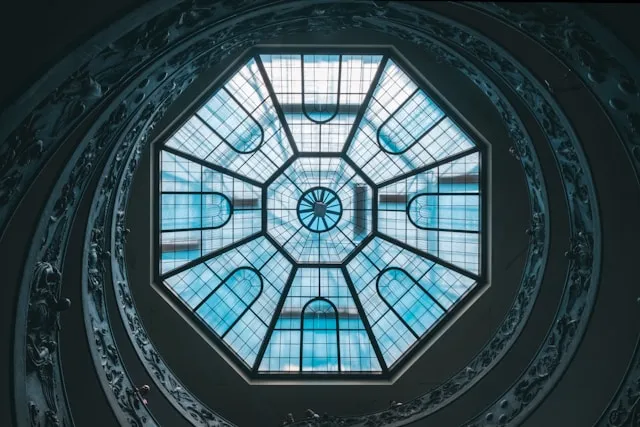
Many ask me “Is Node.js secure?” with the aim of comparing the Node.js runtime with Rust, Go, Java or maybe Deno and Bun. The answer though is a bit more complex than a simple yes or no.
Node.js security is a complex topic. While the core runtime itself has a well-defined threat model that excludes certain attack vectors (like prototype pollution due to JavaScript language structure), it relies on secure coding practices and a layered security approach to mitigate various risks.
The Node.js Threat Model
The Node.js threat model outlines the boundaries of the runtime’s responsibility for security. It defines the scope of vulnerabilities that Node.js itself is responsible for addressing, as well as those that are primarily the responsibility of application developers or other components.
So while the Node.js runtime is designed to be secure in its core functionalities, it also assumes other components and some behavior like the underlying operating system and third-party modules are secure as well, which is not always the case.
Node.js is designed with security controls in mind and being resistant to certain types of vulnerabilities, but it still primarily trusts userland code (the developer’s code) and good coding practices. Think of it like a sturdy house: even if the foundation is strong, the walls, roof, and furnishings can still be vulnerable if they’re not built or maintained properly.
As a practical code reference in Node.js core, I recently provided a patch to address a prototype pollution vulnerability in the child_process
core module:
validateArgumentsNullCheck(args, 'args');if (options === undefined) options = kEmptyObject;else validateObject(options, 'options');
options = { __proto__: null, ...options };let cwd = options.cwd;
// Validate the cwd, if present.if (cwd != null) { cwd = getValidatedPath(cwd, 'options.cwd');}// Validate detached, if present.if (options.detached != null) { validateBoolean(options.detached, 'options.detached');}
This PR adds a (failing) test case that confirms the issue and follows-up with a fix for the bug in child_process
functions to ensure consistent behavior. However, this is not considered a security vulnerability by the Node.js project because the Node.js threat model does not recognize prototype pollution as a viable security vulnerability in the runtime.
Developers play a crucial role in Node.js security. They’re responsible for writing secure code, validating user input, and handling errors correctly. Likewise, third-party modules installed and imported via the npm registry can introduce security risks. Many Node.js applications rely on external libraries and packages to build their applications. While these can save you time and effort, they can also introduce vulnerabilities if they’re not well-maintained or have security flaws.
In a prior article I also explored the Node.js Threat Model and Permissions Model for a broader understanding of how Node.js handles security and publishing Node.js security releases.
Denial of Service (DoS) Attacks in Node.js
Node.js’s single-threaded event loop model, while efficient for many use cases and providing optimal performance results for I/O bound applications, can be susceptible to denial-of-service (DoS) attacks.
A well-crafted request, such as one with a complex regular expression, can overload the event loop, rendering the application unresponsive.
const http = require('http');
http.createServer((req, res) => { // Handle the request, potentially involving a complex regular expression // or computationally intensive task // e.g:
const regex = /([a-z]+)+$/; const input = 'aaaaaaaaaaaaaaaaaaaaaaaa!';
if (regex.test(input)) { res.end('Matched'); } else { res.end('No match'); }
res.end('Hello, world!');}).listen(3000);
As a developer, responsible for userland code, you’d want to put security controls that mitigate the risk of DoS attacks in a Node.js application. For example, considering the following:
- Rate Limiting: Implement rate limiting to restrict the number of requests per unit of time. For example, consider using an SDK such as one provided by Arcjet which adds middlewares to your web framework to rate limit requests.
- Timeout Mechanisms: Set appropriate timeouts for requests to prevent long-running operations from blocking the event loop. For example, consider watchdog mechanism around RegEx operations and running them off the main event-loop thread.
- Asynchronous Operations: Whenever possible, use asynchronous operations to avoid blocking the event loop. For example, consider using the
worker_threads
module to offload CPU-intensive tasks to worker threads.
đź‘‹ Just a quick break
I'm Liran Tal and I'm the author of the newest series of expert Node.js Secure Coding books. Check it out and level up your JavaScript
Node.js Path Traversal Vulnerabilities
Path traversal vulnerabilities can occur when untrusted input is insecurely processed by the application to generate file system paths. This can lead to an attacker accessing files outside the intended directory, potentially exposing sensitive information or executing malicious code.
import fs from 'fs';import path from 'path';
const pathPrefix = '/var/www/uploads/';const userProvidedImagePath = '../../../../etc/passwd';
const imagePath = path.join(pathPrefix, userProvidedImagePath);
// Insecurely evaluating user-provided inputfs.readFile(imagePath, (err, data) => { if (err) { console.error(err); } else { res.send({ image: data.toString('base64') }); }});
Secure Coding Practices in Node.js
Secure coding practices are indispensable for Node.js developers and provide the first-line of defense in their applications from a myriad of vulnerabilities. By adhering to these practices, developers can significantly reduce the risk of attacks, protect sensitive data, and maintain the integrity of their applications.
Consider aspects such as the following to improve the security of your Node.js applications:
- Input Validation: strict validation of user input to prevent malicious data from entering your application, and sanitize input to remove potentially harmful characters or code.
- Output Encoding: encode output data to prevent cross-site scripting (XSS) attacks, code injection and other forms of injection attacks.
- Safe and secure use of JSON parsing and deep cloning to prevent prototype pollution attacks.
- Avoiding code serialization completely (e.g:
new Function()
,eval()
and other forms of dynamic code execution) to prevent code injection attacks.
Conclusion
In conclusion, the question on whether Node.js is a secure runtime or not is a nuanced and multifaceted topic. It’s not just about the core runtime, but also about how the runtime is used - for example: are you referring to using Node.js as a CLI, a desktop application (hi Electron!), or a web application? Each of these use cases has its own security considerations.
In addition, the security of your Node.js applications depends on the code you write, the third-party modules you use, and your awareness of the latest threats. By understanding these factors and taking appropriate measures, you can build secure Node.js applications.