~ 5 min read
Flawed Git Promises Library on npm Leads to Command Injection Vulnerability
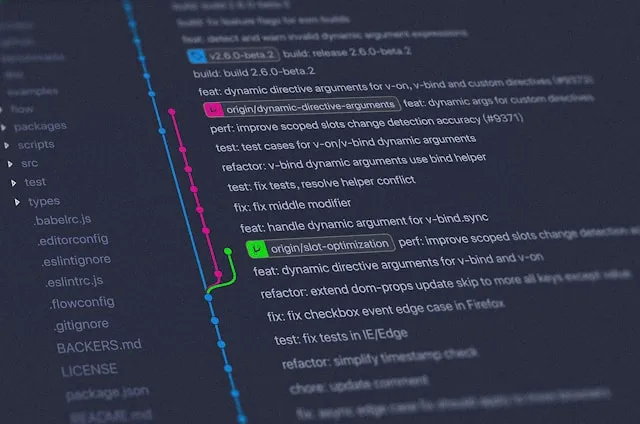
Local promise-returning git command wrappers are popular to see on npm. They provide a convenient way to interact with git
repositories from Node.js applications. However, many of these libraries might be insecure and vulnerable to command injection attacks. The problem is, to know that, you need to either scan it with an SCA tool like Snyk or dive into the source code yourself to understand how it works and whether it harbors any insecure code that no one found yet.
In this article I’ll do that deep-dive I told you about and show you a flawed git promises library on npm that leads to a command injection vulnerability.
Meet ggit
- It’s a simple library that wraps git commands in promises, making it easier to work with git repositories from Node.js applications. The library still gets more than 5,000 weekly downloads on npm, and it’s been around for a while.
Insecure Coding in Git libraries
Consider the following source code in the src/commit-numstat.js
file of the library, which is externally consumable to users of the ggit
library:
var la = require('lazy-ass')var check = require('check-more-types')var exec = require('./exec')var { parseNumstat } = require('./commit-numstat-utils')
function commitNumstat (hash) { la(check.unemptyString(hash), 'missing commit hash', hash)
var cmd = 'git show --numstat ' + hash return exec.exec(cmd).then(parseNumstat)}
module.exports = commitNumstat
Does anything strike you as insecure coding? How about that git
command line build up using the cmd
variable that concatenates user input (the hash
value) into a shell interpreter?
In fact, this is not the only insecure code. Let’s look at src/commit-message.js
for the purpose of performing a git commit
action:
var Q = require('q')var exists = require('fs').existsSyncvar read = require('fs').readFileSyncconst join = require('path').joinvar gitFolder = require('./git-folder')var exec = require('./exec')var la = require('lazy-ass')var is = require('check-more-types')var debug = require('debug')('ggit')
function currentCommitMessage () { debug('getting current commit message')
return gitFolder() .then(root => join(root, '.git', 'COMMIT_EDITMSG')) .then(filename => { if (!exists(filename)) { return Q.reject(new Error('Cannot find file ' + filename)) }
var text = read(filename, 'utf8') /* jshint -W064 */ return Q(text.trim()) })}
/* output of command git show --format="%ae%n%s%n%b" --no-patch <sha> is something like email subject body (optional)
this method returns object with these fields*/function parseCommitMessage (output) { la(is.unemptyString(output), 'expected "git show" command output') const lines = output .split('\n') .map(s => s.trim()) .filter(is.unemptyString) la( lines.length >= 2, 'commit message should at least have email and subject', output ) const body = lines.length > 2 ? lines.slice(2).join('\n') : null return { email: lines[0], subject: lines[1], body: body }}
function commitMessageFor (sha) { la(is.unemptyString(sha), 'expected commit sha', sha) debug('getting commit message for', sha) const cmd = 'git show --format="%ae%n%s%n%b" --no-patch ' + sha return exec.exec(cmd).then(parseCommitMessage)}
function commitMessage (sha) { if (sha) { return commitMessageFor(sha) } return currentCommitMessage()}
module.exports = { commitMessage: commitMessage, commitMessageFor: commitMessageFor, parseCommitMessage: parseCommitMessage}
if (!module.parent) { const sha = process.argv[2] console.log('demo for commit', sha) commitMessage(sha).then(console.log, console.error)}
That’s a lot of code but maybe you already see the problem?
Focus on the commitMessageFor(sha)
function. It builds up a git show
command using the sha
value, which is user input. This is a classic example of a command injection vulnerability.
How would we exploit it? Let’s say we have a sha
value that is a valid git commit hash, but also contains a shell command. For example, sha = '1234567890; echo "exploited"'
. The git show
command would be built up as git show --format="%ae%n%s%n%b" --no-patch 1234567890; echo "exploited"
, which would execute the echo
command after the git show
command.
This is happening because of several things, but more specifically, the exec.exec(cmd)
function in the commitMessageFor
function is using child_process.exec
to execute the cmd
command. This function is known to not be a smart choice to spawn off system commands for anything risky, as it uses the system’s shell interpreter turned on to execute the command.
The ggit
Library Vulnerability
So the finding is that ggit
across all of its versions are known to be vulnerable. The latest version of ggit
is 2.4.12
and hasn’t been fixed nor issued a new security fix version.
2 CVEs have been issued for ggit
that you should be aware of, both are not fixed:
- CVE-2024-21533: Arbitrary Argument Injection
- CVE-2024-21532: Command Injection
Command Injection Best Practices
To avoid command injection vulnerabilities in Node.js code (and likely in other programming languages too), you should follow secure coding conventions, such as:
- Avoid using shell commands: Realistically, you should just opt-out of running system commands. Whenever possible, avoid using shell commands to execute system commands. Instead, use the built-in interfaces and library binding.
- Rely on spawn over exec: If you must run system commands, use
child_process.spawn
orchild_process.execFile
instead ofchild_process.exec
. Thespawn
function does not use the system shell to execute the command, which makes it less prone to command injection attacks. It also uses an array for the command and arguments, which makes it easier to avoid command injection. - Validate user input: Always validate user input. In the case of the
sha
value, you should validate that it is a valid git commit hash and nothing else. As you can see in theggit
library example, that user input of a hash isn’t even validated to conform an expected type such as only alphanumeric characters.
There are other risks and insecure coding conventions you might be falling into when relying on spawning system commands in Node.js, so I wrote a comprehensive and dedicated secure coding book for Node.js developers: Node.js Secure Coding: Defending Against Command Injection Vulnerabilities that I recommend you to read.