~ 4 min read
Bypassing SSRF Protection in nossrf: When Your Safeguards Become Loopholes
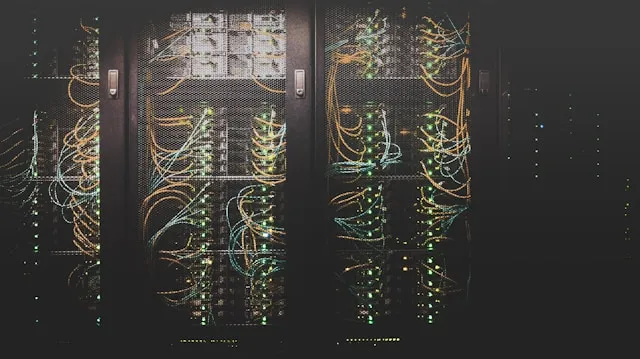
Hey security enthusiasts! Today we’ll take a dive into a critical vulnerability in a popular npm package called nossrf
. This package aims to shield applications from Server-Side Request Forgery (SSRF) attacks by validating user-provided URLs. However, a clever bypass technique renders these safeguards ineffective. Let’s dissect the issue and understand how to stay protected.
What’s SSRF and Why Does nossrf
Matter?
SSRF vulnerabilities arise when an application makes unintended requests to external servers based on user-controlled input. Attackers can leverage this to trick the application into fetching internal resources, performing unauthorized actions, or even launching denial-of-service attacks.
About nossrf
: A Security Layer for SSRF Mitigation
nossrf
steps in by supposedly validating URLs or hostnames before allowing such requests. This offers a valuable security layer for developers building applications susceptible to SSRF attacks.
The Vulnerability: A Flaw in the URL Validation Logic
The crux of the vulnerability lies in nossrf
’s reliance on a specific validation approach. The package utilizes Google’s DNS services to resolve hostnames. While it checks for a successful resolution, it fails to verify a crucial aspect: whether the resolved IP address belongs to a local or reserved IP space. This oversight creates a bypass window for malicious actors.
Here’s the technical breakdown (for our security-savvy readers):
// Resolve hostname using Google's DNS APIconst asyncResolveHostnameGoogle = (async (hostname) => { try { const responseV4 = await axios.get( `https://dns.google/resolve?name=${hostname}&type=A` ); const responseV6 = await axios.get( `https://dns.google/resolve?name=${hostname}&type=AAAA` );
const ips = []; const responses = [responseV4.data, responseV6.data]; for (const response of responses) { if (response.Answer) { ips.push(...response.Answer.map((answer) => answer.data)); } } if(responses[0].Comment) return true if(!responses[0].Comment) return false return ips; } catch { return []; }});
As you can see, the code merely checks for the presence of a Comment
field in the response. If it exists, the IP address is assumed to be safe. This logic is flawed because an attacker can craft a hostname that resolves to a local IP address (e.g., 127.0.0.1
) or a reserved IP range. In such cases, the validation would still pass, even though the request could potentially trigger an SSRF attack.
In simpler terms: nossrf treats any resolved IP address as legitimate, unintentionally opening a door for attackers to exploit.
SSRF Proof of Concept
All versions of nossrf
, up to and including 1.0.3
, are susceptible to this vulnerability.
Here’s a Proof of Concept (PoC) to illustrate the bypass (For educational purposes only!):
- Install the
nossrf
package:
npm install nossrf
- Define an
app.js
file with the programmatic API ofnossrf
as follows:
const nossrf = require("nossrf")const main = async()=>{ let is_safe = await nossrf.asyncValidateUrl("https://google.com") // true means good console.log(is_safe)
let is_safe2 = await nossrf.asyncValidateUrl("https://localtest.me") // true means good console.log(is_safe2)}
main()
- Run the
app.js
file:
node app.js
- Validate that the
localtest.me
hostname resolves to a local IP address space127.0.0.1
and the SSRF protection mechanism is bypassed since for both of the two URLs the response istrue
This PoC demonstrates how a seemingly harmless hostname (localtest.me
) could be used to bypass the SSRF protection. In a real attack scenario, the attacker would craft a hostname resolving to a local IP address on your server, potentially leading to unauthorized actions.
đź‘‹ Just a quick break
I'm Liran Tal and I'm the author of the newest series of expert Node.js Secure Coding books. Check it out and level up your JavaScript
Mitigating the Risk: Patching and Beyond
The most immediate solution is to upgrade to the latest version of nossrf
once a patched version is released. The maintainers will likely address the validation logic to ensure it properly identifies and rejects local or reserved IP addresses.
Here are some additional security best practices to consider:
- Implement input sanitization: This involves filtering out potentially harmful characters or patterns from user-provided URLs.
- Define allowed domains: Restrict valid URLs to specific domains or IP ranges to minimize attack vectors.
- Employ additional security measures: Consider leveraging framework-specific functionalities or libraries that offer deeper SSRF protection mechanisms.
- Remember: Defense in depth is key. Combining various security measures creates a more robust barrier against potential SSRF attacks.
SSRF in particular has more fine-tuned security controls to be implemented to avoid issues such as SSRF via Redirect, SSRF via DNS Rebinding, and SSRF via HTTP Parameter Pollution.
Stay vigilant, and code securely!